Supercharge your workflow
Free online tools for developers, designers, and content creators. No watermarks - just powerful utilities at your fingertips.
Why Choose AtomDyno?
Powerful tools designed to streamline your workflow and boost productivity
Blazing Fast
Optimized tools that deliver instant results without compromising quality.
Intuitive Design
Clean interfaces that make complex tools simple for everyone to use.
Privacy Focused
Your data never leaves your browser. No tracking, no storage.
Dark Mode
Designed for night owls with easy-on-the-eyes dark themes.
Trusted by Developers Worldwide
Join thousands of professionals who rely on AtomDyno for their daily workflow
"AtomDyno Tools has revolutionized my workflow. The image optimization tools saved me hours of work last week alone!"
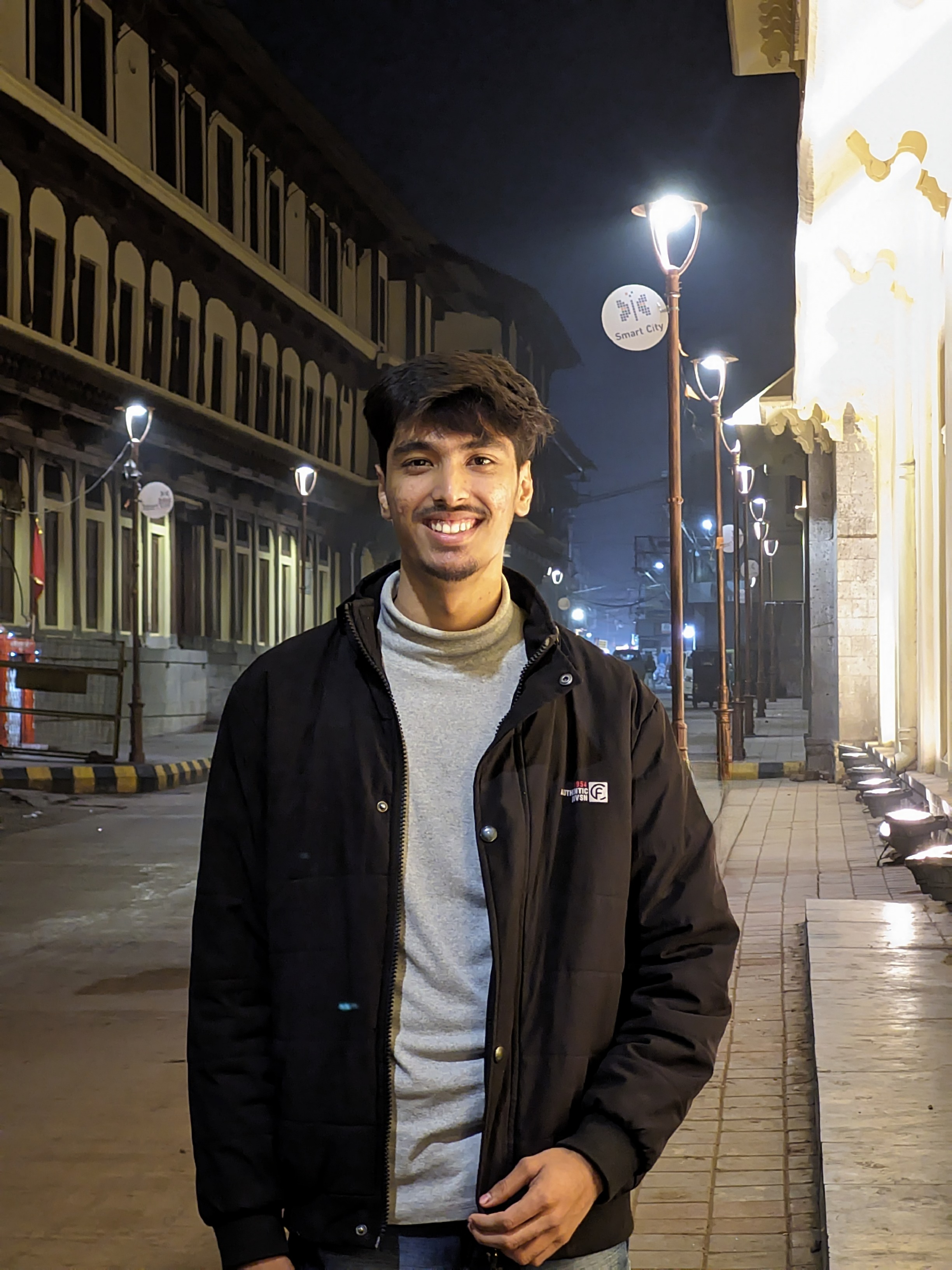
Shourya Gupta
Web Developer
"The PDF tools are incredibly reliable. I use them daily for client deliverables without any quality loss."
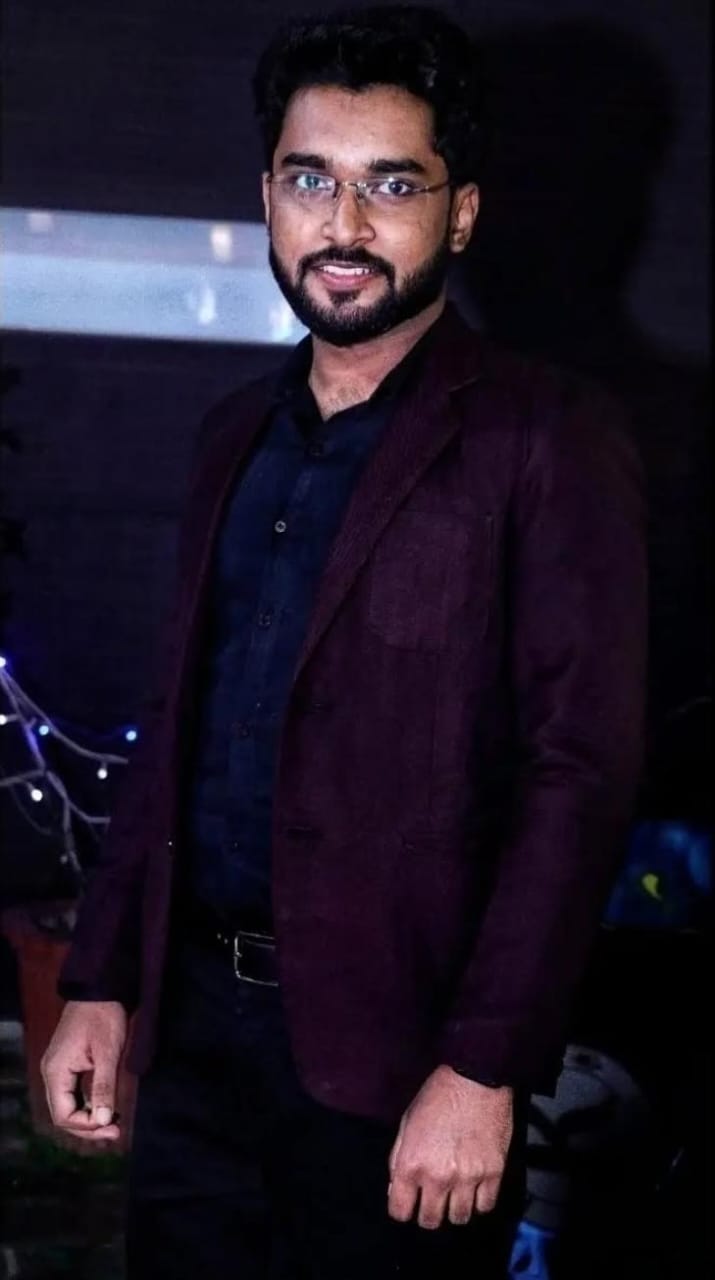
Ayush Sinha
UX Designer
"As a developer, I appreciate tools that just work. AtomDyno's JSON formatter is my go-to for debugging APIs."
Niraj
Full-stack Engineer
Frequently Asked Questions
Find answers to common questions about AtomDyno Tools
Yes, all tools on AtomDyno are completely free to use with no hidden charges. We believe in providing valuable resources to everyone without barriers.
Didn't find what you're looking for?